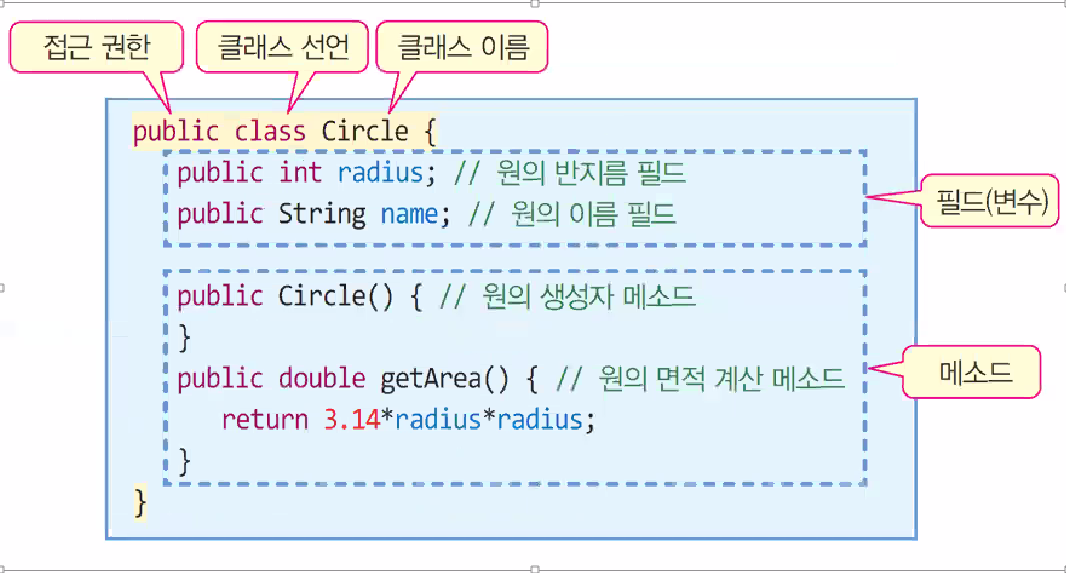
1) 반지름과 이름을 가진 Circle 클래스를 작성하고, Circle 클래스의 객체를 생성하세요.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | package test; public class Circle { // TODO Auto-generated method stub int radius; // 속성 만듬 -> radius변수 String name; // 속성 만듬 public Circle() { // 생성자 } public double getArea() { return 3.14*radius*radius; } public static void main(String[] args) { Circle pizza = new Circle(); // 원 객체 생성 pizza.name = "자바피자"; // 피자 이름설정 pizza.radius=30; // 피자 반지름 설정 double area = pizza.getArea(); // 피자 면적 알기 System.out.println(pizza.name + "의 면적은 "+area ); Circle donut = new Circle(); donut.name="도넛"; donut.radius=10; area = donut.getArea(); System.out.println(donut.name + " 의 면적은 " + area); } } | cs |

2) 너비와 높이를 입력받아 사각형의 합을 출력하는 프로그램을 작성하세요. 너비와 높이 필드, 그리고 면적 값을 제공하는 getArea() 메소드를 가진 Rectangle 클래스를 만들어 활용하세요.
방법1
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | package test; import java.util.Scanner; public class Rectangle { int width; int height; public int getArea() { return width*height; } public static void main(String[] args) { // Rectangle 인스턴스 생성 Rectangle rect = new Rectangle(); // 객체 생성 Scanner sc = new Scanner(System.in); rect.width = sc.nextInt(); rect.height = sc.nextInt(); System.out.println("사각형의 면적은 " + rect.getArea()); sc.close(); } } | cs |
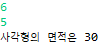
방법 2
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | package test; import java.util.Scanner; public class Rectangle { int width; int height; String name; public Rectangle() { // 생성자 System.out.println("면적을 출력하기 위해 width, height를 입력하세요."); this.width=10; this.height=10; } public int getArea() { return width*height; } public static void main(String[] args) { // Rectangle 인스턴스 생성 Rectangle rect = new Rectangle(); // 객체 생성 Scanner sc = new Scanner(System.in); //rect.width = sc.nextInt(); //rect.height = sc.nextInt(); System.out.println("사각형의 면적은 " + rect.getArea()); sc.close(); } } | cs |

방법 3
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | package test; import java.util.Scanner; public class Rectangle { int width; int height; String name; public Rectangle() { // 생성자 System.out.println("면적을 출력하기 위해 width, height를 입력하세요."); //this.width=10; //this.height=10; } public int getArea() { return width*height; } public static void main(String[] args) { // Rectangle 인스턴스 생성 Rectangle rect = new Rectangle(); // 객체 생성 Scanner sc = new Scanner(System.in); rect.width = sc.nextInt(); rect.height = sc.nextInt(); System.out.println("사각형의 면적은 " + rect.getArea()); sc.close(); } } | cs |

'programming > JAVA' 카테고리의 다른 글
GUI 기초 (1) | 2021.04.30 |
---|---|
db에 파일 넣기 (0) | 2021.04.28 |
JAVA [Scanner 조건문] (0) | 2021.03.23 |
JAVA [Scanner 실습편1] (0) | 2021.03.23 |
JAVA [Scanner] (0) | 2021.03.23 |